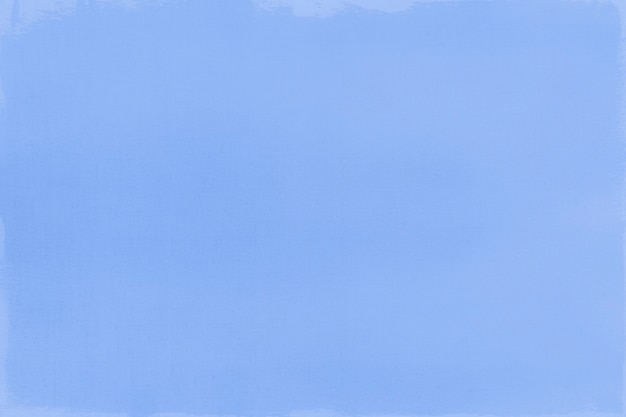
Algorithmic trading brings discipline, precision and speed to market execution that human traders simply can't match. This step-by-step guide will help you develop, test, and implement your first algorithmic trading strategy even if you're new to programming or quantitative methods.
Why Build Your Own Algorithmic Trading Strategy?
While many platforms now offer pre-built strategies, developing your own algorithmic trading system provides several key advantages:
- Customization: Tailor strategies to your specific risk tolerance, time horizon, and market outlook
- Understanding: Gain deeper insights into what drives market movements and strategy performance
- Flexibility: Quickly adapt to changing market conditions by modifying your own code
- Intellectual property: Create a proprietary edge that isn't available to other traders
Perhaps most importantly, building your own algorithms forces rigorous thinking about markets, leading to better trading decisions even when executing manually.
Setting Up Your Development Environment
Before diving into strategy development, you'll need to set up the right tools. Python has become the dominant language for algorithmic trading due to its simplicity, extensive libraries, and strong community support.
Essential Python Libraries
- NumPy and Pandas: Data manipulation and numerical calculations
- Matplotlib/Plotly: Visualization of price data and results
- Scikit-learn: Machine learning algorithms for advanced strategies
- Backtrader or PyAlgoTrade: Backtesting frameworks to evaluate strategy performance
- ccxt: API connections to cryptocurrency exchanges (if trading digital assets)
Development Setup
We recommend using Jupyter Notebooks for strategy development as they allow interactive testing and visualization. For a complete development environment, install Anaconda, which includes Python, Jupyter, and many required libraries.
"Writing an algorithm is a journey of continuous improvement. Start simple, test thoroughly, and iterate based on results rather than aiming for complexity from the beginning."- Jane Street, Quantitative Researcher
Choosing Your First Strategy Type
For your first algorithmic strategy, start with a well-established approach that's conceptually simple:
Moving Average Crossover
This strategy generates signals when a faster moving average crosses a slower one. Despite its simplicity, it captures trends effectively and serves as an excellent starting point.
Sample Python Implementation
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
# Load your price data into a DataFrame
df = pd.read_csv('price_data.csv')
# Calculate moving averages
df['MA_Fast'] = df['Close'].rolling(window=20).mean()
df['MA_Slow'] = df['Close'].rolling(window=50).mean()
# Generate signals
df['Signal'] = 0
df.loc[df['MA_Fast'] > df['MA_Slow'], 'Signal'] = 1 # Buy signal
df.loc[df['MA_Fast'] < df['MA_Slow'], 'Signal'] = -1 # Sell signal
# Calculate daily returns
df['Returns'] = df['Close'].pct_change()
# Calculate strategy returns
df['Strategy_Returns'] = df['Signal'].shift(1) * df['Returns']
Mean Reversion
These strategies assume prices tend to revert to their mean over time. A simple version might buy when prices move significantly below a moving average and sell when they move above it.
Trend Following
Beyond simple moving averages, more sophisticated trend-following systems use indicators like ADX (Average Directional Index) to identify strong trends before entering positions.
Data Collection and Preparation
High-quality data is the foundation of any successful algorithmic strategy:
Data Sources
- Alpha Vantage: Free API with historical and real-time data
- Yahoo Finance: Free historical data through the yfinance Python package
- Interactive Brokers: High-quality data if you have an account
- Quandl: Extensive financial and economic datasets (some free, some premium)
Data Cleaning and Preparation
Before using data for strategy development, ensure you:
- Handle missing values appropriately
- Adjust for splits and dividends if using equity data
- Normalize data if using multiple instruments
- Check for outliers that could skew backtesting results
Backtesting Your Strategy
Backtesting simulates how your strategy would have performed using historical data. While no guarantee of future performance, it's essential for evaluating and refining your approach.
Key Backtesting Considerations
- Transaction costs: Include realistic commission and slippage estimates
- Look-ahead bias: Ensure your strategy only uses information available at the time of each decision
- Survivorship bias: When testing on stocks, include delisted companies
- Overfitting: Test on out-of-sample data to ensure your strategy isn't just curve-fitted to historical patterns
Performance Metrics
Don't just focus on total returns. Evaluate your strategy using these additional metrics:
- Sharpe Ratio: Returns relative to risk (volatility)
- Maximum Drawdown: Largest peak-to-trough decline
- Win Rate: Percentage of profitable trades
- Profit Factor: Gross profits divided by gross losses
- Calmar Ratio: Returns relative to maximum drawdown
Position Sizing and Risk Management
Even the best entry and exit signals need proper position sizing to create a robust strategy:
Fixed Percentage Risk
Risk a consistent percentage of your portfolio on each trade, adjusting position size to ensure consistent risk exposure.
def calculate_position_size(account_value, risk_percentage, entry_price, stop_loss_price):
"""
Calculate position size based on fixed percentage risk
"""
# Calculate dollar risk amount
dollar_risk = account_value * risk_percentage
# Calculate per-unit risk
per_unit_risk = abs(entry_price - stop_loss_price)
# Calculate position size
position_size = dollar_risk / per_unit_risk
return position_size
Volatility-Based Position Sizing
Adjust position sizes based on market volatility, reducing exposure during turbulent periods.
Correlation Management
If trading multiple instruments, account for correlations to avoid unknowingly concentrating risk.
Moving from Backtest to Live Trading
The transition from backtesting to live trading requires careful preparation:
Paper Trading
Before risking real capital, implement your strategy in a paper trading environment to ensure it works as expected in real-time conditions.
API Selection
Choose a broker with a reliable API that supports algorithmic trading. Popular options include:
- Interactive Brokers (comprehensive API with global market access)
- Alpaca (commission-free API designed specifically for algorithmic trading)
- TD Ameritrade (solid REST API with good documentation)
Infrastructure Considerations
Determine where your algorithm will run:
- Local machine: Simplest but potentially unreliable
- Cloud server: More reliable but requires additional setup
- Broker-provided solutions: Some brokers offer hosting for algorithms
Debugging and Common Issues
Be prepared for these common challenges when implementing your first algorithm:
- Data issues: Inconsistent formats, missing values, or incorrect adjustments
- Look-ahead bias: Accidentally using future information in your trading decisions
- Over-optimization: Creating a strategy that works perfectly on historical data but fails in live trading
- Execution discrepancies: Differences between expected and actual execution prices
Maintaining and Improving Your Algorithm
Algorithmic trading requires ongoing maintenance and improvement:
- Regularly review performance metrics and compare to expectations
- Set up automated monitoring and alerts for unexpected behavior
- Establish a systematic approach to refining parameters without overfitting
- Document all changes and their impacts to build institutional knowledge
Conclusion: Your Algorithmic Trading Journey
Building your first algorithmic trading strategy is just the beginning of a rewarding journey. As you gain experience, you'll develop more sophisticated approaches, incorporate machine learning techniques, and potentially create a portfolio of complementary strategies that work together to generate consistent returns across different market conditions.
Remember that successful algorithmic trading combines technical skill with deep market understanding. Balance your focus between code optimization and developing insights into the fundamental drivers of market behavior. With persistence and continuous improvement, algorithmic trading can provide a powerful edge in today's complex financial markets.